numpy의 특징은 일반 list에 비해 빠르고, 메모리를 효율적으로 사용할 수 있고 반복문 없이 데이터 배열에 대한 처리를 지원한다.
install
activate ml
conda install numpy
import
import numpy as np
array creation
np.array 함수를 사용하여 array를 생성할 수 있다.
test_array = np.array([1, 4, 5, 8], float)
shape, dtype
shape으로 array의 dimension 구성을 확인 할 수 있고 dtype으로 array의 데이터 type을 확인 할 수 있다. 위에서 array를 생성할때 지정해준 float가 바로 dtype을 지정해준 것이다.
test_array.shape
test_array.dtype
nbytes
ndarray object의 메모리 크기를 반환하는 함수이다.
np.array([[1, 2, 3], [4.5, "5", "6"]], dtype=np.float32).nbytes
reshape
array의 shape의 크기를 변경할 수 있는 함수이다.
np.array(test_matrix).reshape(8,)
예를 들어서 reshape(-1, 2)라고 해주면 여기서 -1은 뒤에 2를 기준으로 알아서 숫자를 맞춰달라는 의미가 된다.
flatten
다차원 array를 1차원 array로 반환해준다.
np.array(test_matrix).flatten()
indexing
list와는 달리 이차원 배열에서 [0,0]과 같은 표기법을 제공해준다.
print(test_matrix[0, 0])
print(test_matrix[0][0]) #물론 이렇게도 표기할 수 있다.
slicing
슬라이싱도 list와 달리 행과 열 부분을 나눠서 슬라이싱이 가능하다
print(test_matrix[:, 2:])
print(test_matrix[1, 1:3])
arange
array의 범위를 지정하여 값의 list를 생성하는 함수이다.(가끔 arrange와 헷갈린다....)
np.arange(30)
ones, zeros, empty
#zeros : 0으로 가득찬 ndarray 생성
np.zeros(shape, dtype, order)
#ones : 1로 가득찬 ndarray 생성
np.ones(shape, dtype, order)
#empty : shape만 주어지고 비어있는 ndarray 생성
np.empty(shape=(10,), dtype=np.int8)
something_like
기존 ndarray의 shape 크기만큼 1, 0 또는 empty array를 반환해준다.
np.ones_like(test_matrix)
np.zeros_like(test_matrix)
identity
단위 행렬을 생성해주는 함수이다.
np.identity(5)
eye
identity와 비슷하지만 시작 index를 지정해줄 수 있다.
np.eye(3, 5, k=2)
diag
대각 행렬의 값을 추출해주는 함수이다.
np.diag(matrix)
random sampling
데이터 분포에 따른 sampling으로 array를 생성하는 것을 말한다.
# 균등분포
np.random.uniform(0, 1, 10).reshape(2, 5)
# 정규분포
np.random.normal(0, 1, 10).reshape(2, 5)
sum
element들 간의 합을 구하는 함수이다.
test_array.sum(dtype=np.float)
axis
모든 operation function을 실행할 때 기준이 되는 dimension 축을 의미하며 처음에는 상당히 헷갈리는 부분이다.
test_array.sum(axis=0)
test_array.sum(axis=1)
다음과 같이 그림으로 확인하면 좀 더 이해하기 쉽다.
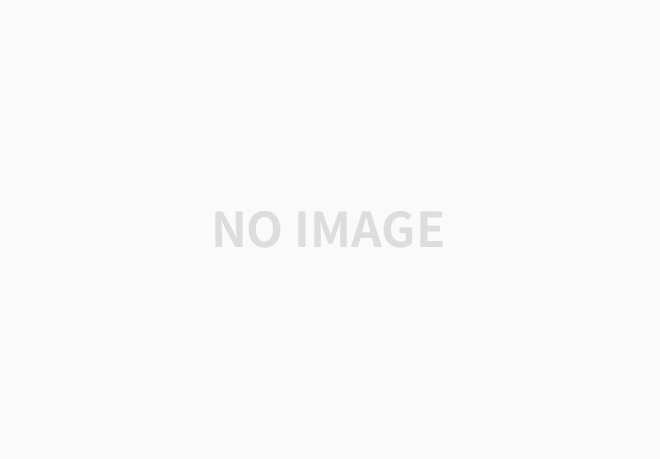
mean & std
각각 평균값과 표준 편차를 반환해주는 함수이다.
# 평균값
test_array.mean()
# 표준편차
test_array.std()
vstack & hstack
vstack에서 v는 vertical일거라고 쉽게 유추할 수 있고 vertical 방향으로 합쳐지는 함수이다. 즉, 행끼리 합쳐진다고 생각하면 된다. 반대로 hstack은 horizontal 방량으로 합쳐지는 함수이므로 열끼리 합쳐지는 함수라 생각하면 된다.
np.vstack((a,b))
np.hstack((a,b))
concatenate
concatenate는 axis를 지정하여 방향을 정하고 array끼리 합쳐주는 함수이다.
np.concatenate((a,b), axis=0)
np.concatenate((a,b), axis=1)
array operations
다음과 같이 array간의 기본적인 사칙 연산을 지원한다.
test_a + test_a
test_a - test_a
test_a * test_a # element 곱이 이루어진다
Dot product
행렬곱은 dot함수를 사용한다.
test_a.dot(test_b)
transpose
전치행렬은 array 뒤에 T 또는 transepose()를 붙여주면 된다.
test_a.T
test_a.transpose()
broadcasting
다음과 같이 두 개의 array의 shape이 다르면 분배법칙에 따른 계산이 이루어진다.
timeit
주피터 환경에서 코드의 퍼포먼스를 체크하기위해 timeit 함수를 사용할 수 있다.
%timeit np.arange(test_a) * scalar
일반 적으로 속도는 다음과 같이 나온다.
for loop < list comprehention < numpy
All & Any
array의 데이터 전부(and) 또는 일부(or)가 조건에 만족하는 여부를 반환해주는 함수들이다.
a = np.arange(10)
np.any(a > 5)
np.any(a < 0)
np.all(a > 5)
np.all(a < 10)
comparison operation
numpy는 배열의 크기가 동일 할 때 element간 비교의 결과를 boolean type으로 반환해준다.
test_a = np.array([1, 3, 0])
test_b = np.array([5, 2, 1])
test_a > test_b
test_a == test_b
a = np.array([1, 3, 0])
np.logical_and(a > 0, a < 3) # and 조건의 condition
b = np.array([True, False, True])
np.logical_not(b) # not 조건의 condition
c = np.array([False, True, Flase])
np.logical_or(b, c) # or 조건의 condition
where
np.where(a > 0, 3, 2) #where(condition ,True, False)
np.where(a > 0) # index 값을 반환
np.isnan(a) # np.nan 값인지 확인
np.isfinite(a) # np.inf 값인지 확인
argmax & argmin
array내 최대값 또는 최솟값의 index를 반환한다.
np.argmax(a)
np.argmin(a)
# axis 기반으로 반환
np.argmax(a, axis=1)
np.argmin(a, axis=0)
boolean index
특정 조건에 따른 값을 배열 형태로 추출해줄 수 있다.
test_array[condition > 3] # True인 값의 위치 index에 맞는 element만 추출된다
fancy index
numpy는 array를 index value로 사용해서 값을 추출할 수 있다.
a = np.array([2, 4, 6, 8])
b = np.array([0, 0, 1, 3, 2, 1]) # 반드시 integer로 선언
a[b] # bracket index, b 배열의 값을 index로 하여 a의 값들을 추출
a.take(b) #bracket index와 같은 효과
loadtxt & savetxt
text type의 데이터를 읽고 저장하는 함수들이다.
a = np.loadtxt("./test.txt")
np.savetxt('test.csv', a, delimiter=',')
'Boostcamp AI Tech' 카테고리의 다른 글
[Boostcamp Day-5] Python - Pandas (0) | 2021.08.06 |
---|---|
[Boostcamp Day-5] Python - Data Structure (0) | 2021.08.06 |
[Boostcamp Day-5] Python - Data Handling (0) | 2021.08.06 |
[Boostcamp 과제 - 5] morsecode (0) | 2021.08.06 |
[Boostcamp 과제 - 4] Baseball (0) | 2021.08.06 |